Bandit13
Website URL: http://overthewire.org/wargames/bandit/bandit13.html
Log in to the game server using credentials of bandit12.
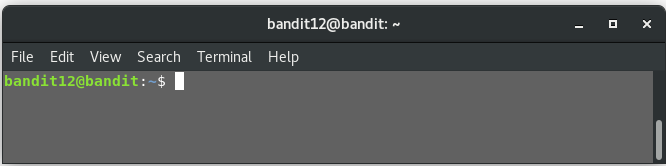
Our task for bandit13:
The password for the next level is stored in the file data.txt, which is a hexdump of a file that has been repeatedly compressed. For this level it may be useful to create a directory under /tmp in which you can work using mkdir. For example: mkdir /tmp/myname123. Then copy the datafile using cp, and rename it using mv (read the manpages!)
Let’s have a look at the contents of the file.
cat data.txt
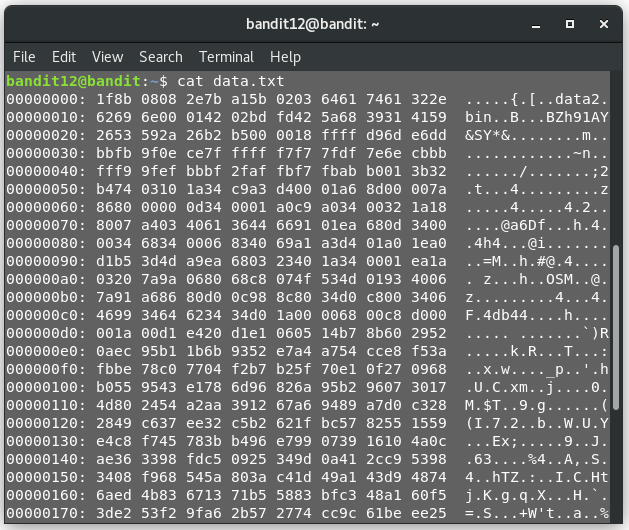
As told in the file, this seems to be the raw output of a hexeditor/hexdump. The first thing we should do, is to reconstruct the orginal file from this hexdump.
Now we don’t have WRITE access in the home directory. So in order to make files and scripts (and we may be required to do that a lot in subsequenct challenges) we need to make a directory in /tmp folder, where we get the write access. Let’s switch working to that directory. I am using the directory name as solvingBandit2018.
mkdir /tmp/solvingBandit2018
cp data.txt /tmp/solvingBandit2018
cd /tmp/solvingBandit2018
ls
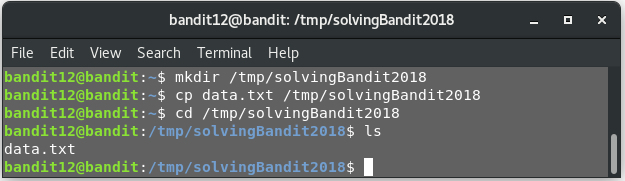
Now, we can get down to working on getting the required password. Let’s see what kind of file we are given.
file data.txt
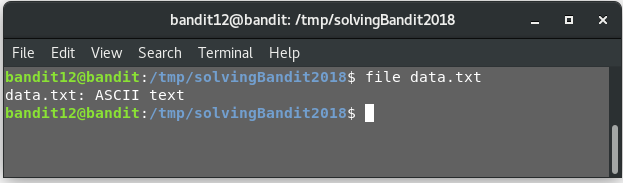
The data.txt file we are provided is just a text file containing the hexdump. So our first step is to recompile the original file from this hexdump. There is a very useful command xxd that can be used for this purpose.
The xxd can generate or reverse hex dumps. In our case we want to perform a reverse operation on data.txt so that we can obtain a compressed file. Now, there can be any of the various compression types. So, initially, lets extract it into a generic file.
xxd -r data.txt compressed
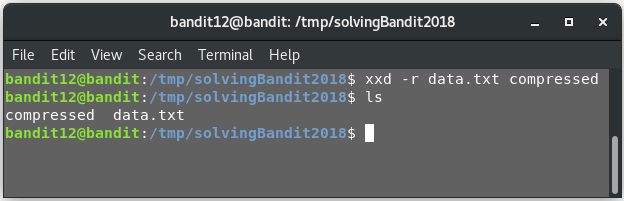
Let’s find out now how the file is compressed.
file compressed
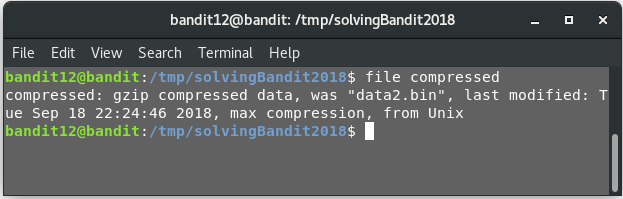
Now, this file is the result of repeated compression, so we need to extract all of those levels. We can either do it manually, which is a guaranteed way to get to the bottom of it, but what I am going to do here is, try to develop a script to extract it in an automated fashion.
Well, although its not guaranteed to work at this point of time, but it’s going to be a fun learning experience!
Now, most of the compressed files fall into one of the following categories:
- gzip
- bzip2
- tar
- zip
Now, let’s try to build a script for getting the files automatically, find the compression type and then decompress it. The script should do this repeatedly until there is no unprocessed compressed file left in the directory. I used python 3 to do this. The code is as follows:
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import os
import subprocess
def get_compression_type(filename):
print ("Getting compression type for file: ", filename)
proc = subprocess.Popen(["file", filename], stdout=subprocess.PIPE)
res = proc.communicate()[0].decode('ascii')
supported_types = ['gzip','bzip2','zip','tar']
for item in supported_types:
if item in res:
print("Found filetype: ",item)
return item
return "NULL"
def get_filenames(filterlist):
flist = []
proc = subprocess.Popen(["ls"],stdout=subprocess.PIPE)
res = proc.communicate()[0].decode('ascii')
fl = res.strip().split('\n')
flist = [x for x in fl if x not in filterlist]
return flist
def decompress(filename):
t = get_compression_type(filename)
cmd = ''
flag = False
print(t)
if t == 'gzip':
p = subprocess.Popen(['mv',filename, filename+'.gz'])
command = 'gzip -dN '+ filename+'.gz'
flag = True
elif t == 'bzip2':
command = 'bzip2 -d '+ filename
flag = True
elif t == 'tar':
command = 'tar -xvf '+ filename
flag = True
elif t == 'NULL':
print("No supported filetype! Exiting")
flag = False
if flag == True:
print ("Executing command: ", command)
proc = os.system(command)
else:
pass
return flag
def main():
init_flist = ['data.txt']
flist = get_filenames(init_flist)
while flist != init_flist:
for item in flist:
f = decompress(item)
if f == True:
init_flist.append(item)
flist = get_filenames(init_flist)
else:
print('Ending Process at latest file: ',item)
init_flist.append(item)
return
return
if __name__ == '__main__':
main()
You can either build a similar (or even a better) script, or use the one above. Put it up in the server (either by creating a file on the server itself, or creating a local file and then transferring it to the bandit12 server) and execute. Let’s look at what happens:
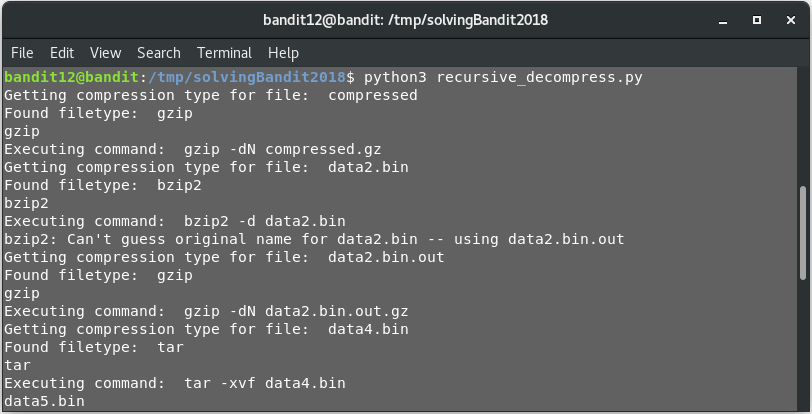
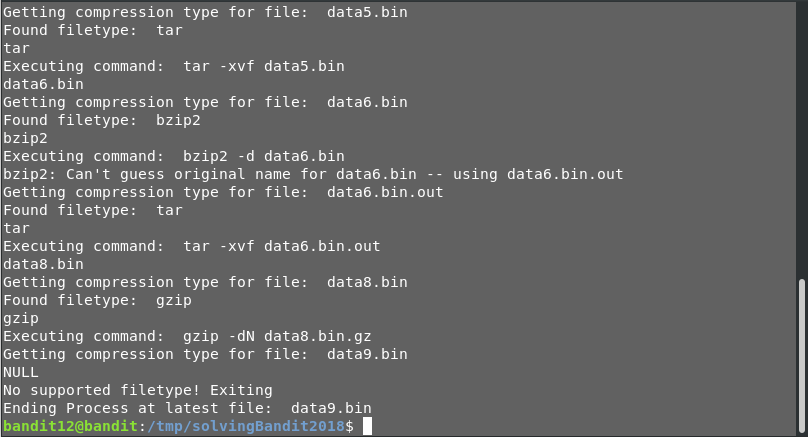
Well, our algorithm seems to have stopped at the file data9.bin. This file doesn’t seem to be any of the supported types we added to our script. Let’s see what type of file this is.
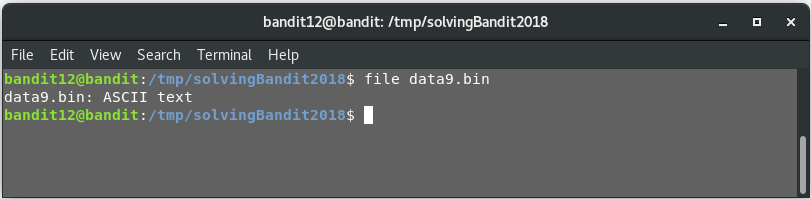
This file is not a compressed file, but just ASCII text. So, let’s see the contents.
Solved it!